In this post, we’ll take you step-by-step through the process of creating and using your own custom shortcodes. We’ll go over what shortcodes are, walk you through the entire process of creating a new shortcode, and show you how to modify and control the shortcode attributes and functions.
Please note that this is a more advanced post that will go into details on how to create shortcodes. If working with code is beyond your technical expertise, and you just want the easiest way to get started with creating and using shortcodes, you may want to start with a shortcode plugin.
A Little History Trip
Did you know that shortcodes were introduced back in WordPress 2.5? That’s right, they’ve been around for quite some time, making lives easier for WordPress users who wanted to add custom content without diving deep into PHP. Initially, shortcodes were a simple way to add macro codes for repetitive content, but over time, they’ve evolved into a powerful tool for website customization.
In short, WordPress shortcodes are used to 1) reduce the amount of code you need to write; 2) simplify the usage of WordPress plugins, themes, and other functions. They behave like macros, when you insert a shortcode, it is replaced with a snippet of code. It could be anything.
WordPress comes with some predefined shortcodes, like ‘gallery’ and ‘caption’ and they’re also included with many popular plugins and themes. You can also create your own custom shortcodes to do things like create columns on your WordPress website.
Setting the Stage for Custom Shortcodes
When creating your own shortcodes, there are two things you need to do:
- Create the shortcode handler function – A shortcode function is a function that takes optional parameters (attributes) and returns a result.
- Register the shortcode handler function – Use the built-in WordPress add_shortcut function to register custom shortcodes.
Preparing WordPress for Custom Shortcodes
Although it’s not required, it’s a good idea to keep your custom shortcodes in their own file. Alternatively, you may add them to your theme’s functions.php file.
First, create a new file named custom-shortcodes.php, and save it inside the same folder as your theme’s functions.php file.
Then, inside the newly created file, add the following block of code:
<?php
?>
Next, open the functions.php file, and add the following line of code:
include('custom-shortcodes.php');
You’re now ready to start adding your custom shortcodes.
Creating Basic Shortcodes
In this first example, we’re going to create a basic WordPress shortcode that inserts the Day Of The Indie avatar image below.
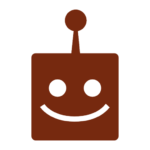
Creating the Shortcode
The first step is to create the shortcode function. Inside the custom-shortcodes.php file, add the following block of code:
function dotiavatar_function() {
return '<img src="http://dayoftheindie.com/wp-content/uploads/avatar-simple.png"
alt="doti-avatar" width="96" height="96" class="left-align" />';
}
In the code example above, the dotiavatar_function function returns a predetermined image named avatar-simple.png.
The next step is to register the shortcode with WordPress using the built-in function add_shortcode. Still inside custom-shortcodes.php, add the following line of code:
add_shortcode('dotiavatar', 'dotiavatar_function');
When you register a shortcode using the add_shortcode function, you pass in the shortcode tag ($tag) and the corresponding function ($func)/hook that will execute whenever the shortcut is used.
In this case, the shortcut tag is dotiavatar and the hook is dotiavatar_function.
Note: When naming tags, use lowercase letters only, and do not use hyphens; underscores are acceptable.
Using the Shortcode
Now that you have the shortcode created and registered, it’s time to try it out! Whenever you want the DOTI avatar to appear inside the post content, you can use the shortcode instead:
[dotiavatar]
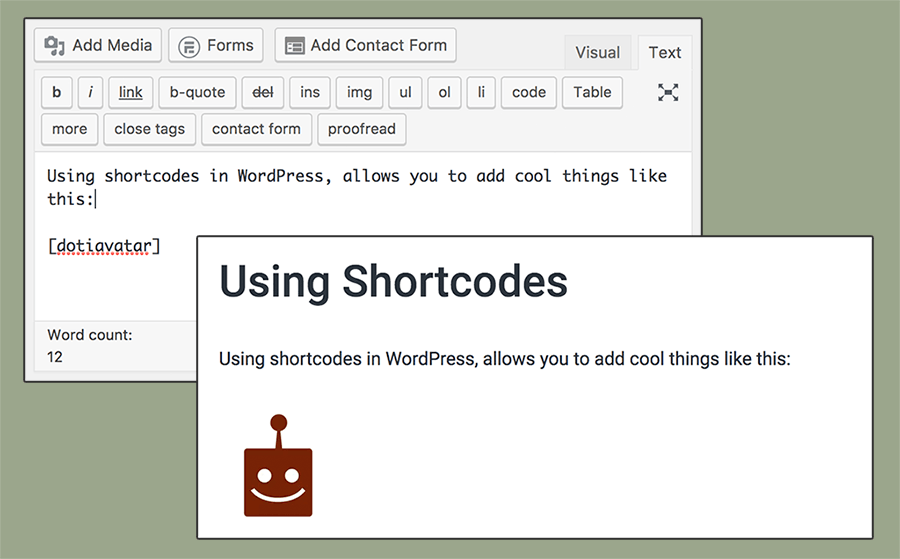
Enhancing Shortcodes with Parameters (Attributes)
In the previous example, there wasn’t much room to change things up. Let’s say, instead of pushing a single image, we’d like to be able to set which image to use using a parameter. You can do that by adding some attributes ($atts).
Once again, inside custom-shortcodes.php, add another function, like so:
function dotirating_function( $atts = array() ) {
// set up default parameters
extract(shortcode_atts(array(
'rating' => '5'
), $atts));
return "<img src="http://dayoftheindie.com/wp-content/uploads/$rating-star.png"
alt="doti-rating" width="130" height="188" class="left-align" />";
}
The function above accepts a single parameter: rating. If a rating value is not passed, it uses a default string value of 5. It does this by unwrapping the array of attributes using the built-in shortcode_atts function, and combining the default values with values that may have been passed into the function.
Don’t forget to register the shortcode:
add_shortcode('dotirating', 'dotirating_function');
With the shortcode function created, and the hook added, the shortcode is now ready to be used inside your post content:
[dotirating rating=3]
Alternatively, you can omit the rating, and just go with the default value:
[dotirating]
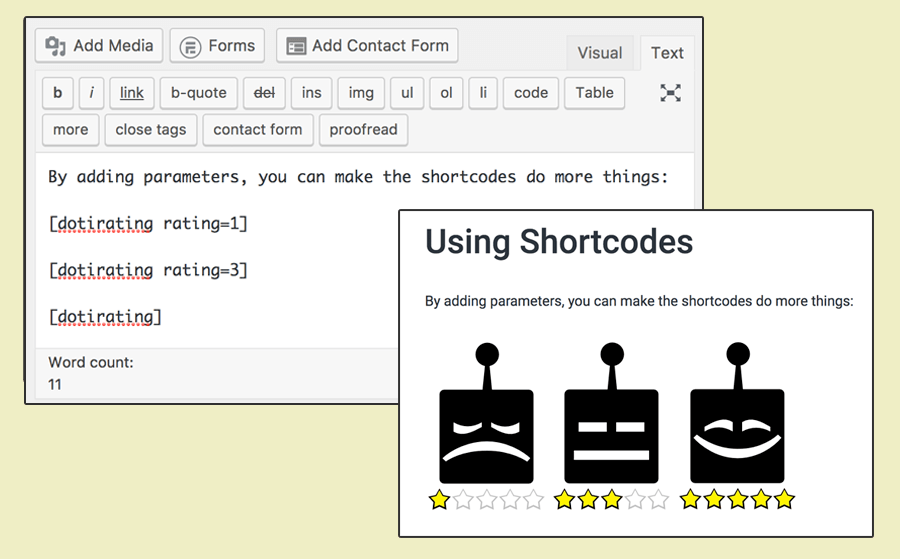
Exploring Enclosing Shortcodes
Up until now, we’ve been working with self-closing shortcodes. But there is another type of shortcode: enclosing shortcodes.
Enclosing shortcodes allows you to use a BBCode-style format. That is, a style that looks like this:
[shortcode]content[/shortcode]
Enclosing shortcodes are useful for when you need to manipulate the enclosed content. For example, let’s say you have a particular style of button you use your website; you could use the HTML code to generate that button/style every time you need to use it, or you can set up a custom enclosing shortcode instead.
By using an enclosing shortcode, you’re able to keep the focus on the content, rather than on the code.
Creating the Shortcode
Again, inside the custom-shortcodes.php file, add the following block of code:
function dotifollow_function( $atts, $content = null ) {
return '<a href="https://twitter.com/DayOfTheIndie" target="blank" class="doti-follow">' . $content . '</a>';
}
In the code block above, the $content = null is used to identify this function as an enclosing shortcode. And, inside that function, you’re wrapping your content ($content) within the HTML code.
OK, now it’s time to register the shortcode:
add_shortcode('dotifollow', 'dotifollow_function');
And that’s it! Your shortcode is ready to be used.
Using the Shortcode
Using an enclosing shortcode isn’t much different than using a self-closing one. Like HTML, you just need to make sure you have an opening and a closing statement:
[dotifollow]Follow us on Twitter![/dotifollow]
Of course, this is just a basic example. You can also add parameters to an enclosing shortcode, like you do with a self-closing shortcode.
Inside custom-shortcodes.php, add one more function:
function dotibutton_function( $atts = array(), $content = null ) {
// set up default parameters
extract(shortcode_atts(array(
'link' => '#'
), $atts));
return '<a href="'. $link .'" target="blank" class="doti-button">' . $content . '</a>';
}
And then register the shortcode:
add_shortcode('dotibutton', 'dotibutton_function');
This new function let’s you set a link for the button using the following syntax:
[dotibutton link="/bits-bytes/"]Shop Now![/dotibutton]
With enclosing shortcodes, you can do a lot with very little code.
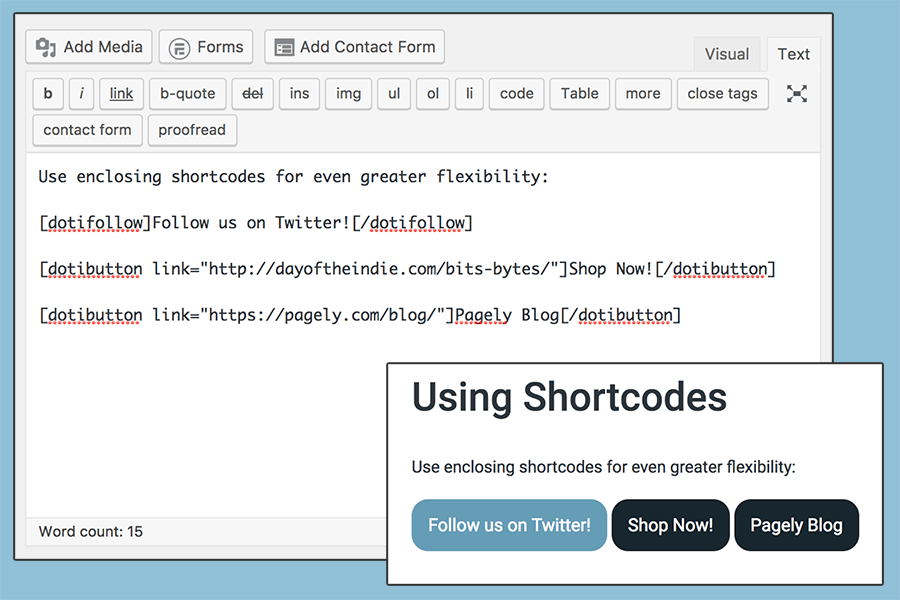
A Word About Widgets
By default, shortcodes are only supported in posts, pages, or custom post types; they are not supported in sidebar widgets. To add support for widgets, you need to add the following code to the functions.php file:
add_filter( 'widget_text', 'shortcode_unautop' );
add_filter( 'widget_text', 'do_shortcode' );
Once you do that, you can use shortcodes in widgets- just like you do in posts/pages.
Debugging Your Shortcodes
Sometimes, despite your best efforts, a shortcode might not behave as expected. It’s like baking a cake – sometimes it just doesn’t rise. When this happens, there are a couple of things you can check:
- Ensure there’s no typo in the shortcode tag or attributes.
- Make sure the shortcode function is correctly included and registered in your theme’s functions.php file or your custom plugin file.
- If the shortcode outputs HTML, verify that the HTML is correctly formatted and not missing any closing tags.
For a deeper dive, you might want to use debugging plugins or check WordPress’s debug mode to catch any underlying issues.
Wrapping Things Up
Adding your own shortcodes doesn’t take a lot of effort- especially once you understand how they’re implemented. If you’re eager to dive deeper into the world of WordPress customization, exploring the official WordPress Codex or seeking out community forums can be a fantastic way to expand your knowledge and skills. Remember, the world of coding is vast and always evolving, so staying curious and continuously learning is key to mastering it.
Thanx, it was exactly what i’m looking for
What about saving the data? either for re-use or copying the shortcode, changing attributes and saving as new shortcode?
Is that possible… I mean to have it with an editor window, where one can save copies of the shortcodes to past into other pages or edit later?
I have a basic setup with 9 attributes, but it get tedious having to retype all them over, and over again, with incremental changes each time
Hi Great job
Easy way to teach thank you so much
This does not work at all. I followed the directions to the letter, and instead of the shortcode calling the PHP function, it just appears in my pages for my users to see, like “[shortcode]”. Great.
Thank you Tammy :*
This was exactly the training I was looking for
Thank you for teaching me so comprehensive. 🙂
Worked first time!
Thanks so much for making it so easy to understand and follow.
This is amazing. Thank you so much!!!!
Hi Tammy
This post is awesome. I have been searching for literally days for a simple explanation and this post is just perfect. I do have a question however (!). Hope that’s ok! Could you add to the above to explain how to load libraries your php code relies on. I have built some awesome php code that runs beautifully on my local web server but it does require some external third party libraries. After reading your post I know I will easily manage to get the code onto my wordpress site but it obviously won’t work unless I also load up the libraries it uses. How do I get those loaded? Where do I put them? And do I just uses regular “require” statement in my functions code? How does it all work?
Great Article – thanx!
thanks for tutorials .
How can we add a button to the editor for this shortcode?
Adding custom shortcodes now a fun game after reading your guide. Thanks, Tammy Coron.
very helpful and efficient, thanks.
Hi,
Great tut.
I am learning WP. I have a custom template page, That has google map and html table under google map. I would like to use this template in many pages on my site. Can I call my custom template page a short code and inject this short code in different places?
Thank you very much, I actually added this module on my site, and glad it worked, otherwise I’ve to remove the [separate] from all (150) pages of my website.
Thanks again Tammy~